Highlights
- Step-by-step setup for Chai environment and API integration.
- Detailed guide on structuring AI conversation flows.
- Tips for training and improving AI response accuracy.
- Testing strategies for reliable chatbot performance.
- Deployment options for publishing and embedding Chai-powered AI.
The field of conversational AI has rapidly evolved, and with tools like Chai, developers can quickly create robust AI-powered chatbots and applications for various scenarios. Whether you’re building a customer service chatbot, an AI-powered recommendation engine, or even a digital companion, Chai provides a platform for easy setup, training, and deployment of conversational AI.
Though you can have our AI & GPT integration services, we articulate this guide, to walk you through how to code use cases for AI using Chai. You’ll learn how to set up Chai, structure conversation flows, train and test your AI, and deploy it for real-world use.
Understanding Chai and AI Use Cases
Chai is a conversational AI platform designed for developing chatbots and virtual assistants that can seamlessly interact with users in natural language. The platform is known for its pre-trained models, APIs, and user-friendly tools, which make it a preferred choice for building interactive AI applications without needing extensive expertise in machine learning or natural language processing (NLP)
A “use case” refers to the specific application or scenario in which an AI can provide value, such as helping users with customer support queries, making product recommendations, or providing personal coaching. By tailoring the AI to handle a particular set of user interactions, you can create a specialized experience that meets user needs effectively. Some key benefits of using the Chai AI chatbot for these use cases include:
Pre-trained Models
Chai’s built-in NLP capabilities allow for faster development by handling the complexities of language understanding.
Flexible API Integration
Chai’s API can integrate with various platforms, making it versatile and applicable to different industries and scenarios.
Focus on Conversation Flows
Chai emphasizes creating engaging, user-oriented interaction flows, ideal for customer-centric applications.
Setting Up Chai for AI Development
To start coding your AI use case with Chai, you’ll first need to set up the development environment and install the necessary packages.
Step-by-Step Setup Guide
Install the Chai Package
Start by installing the Chai package using `pip`, Python’s package manager.
“`bash
pip install chai
“`
Account Setup
If Chai requires user registration, create an account on the platform’s website. You may also need to subscribe to access specific features, so check their requirements.
API Key
Once you’re registered, Chai may provide an API key, which you’ll need to include in your code for authentication. You can obtain this from Chai’s developer dashboard.
Basic Connection Test
To ensure everything is set up correctly, run a simple test to confirm the API connection.
Example Code
“`python
from chai import ChaiClient
client = ChaiClient(api_key=”your_api_key”)
print(“Chai setup successful!”)
“`
This basic test verifies that your environment is connected to Chai’s platform, so you’re ready to start coding.
Defining and Coding Use Cases with Chai
With the environment set up, the next step is to define the AI use case you want to develop and code its interactions.
Step 1: Identify Your Use Case
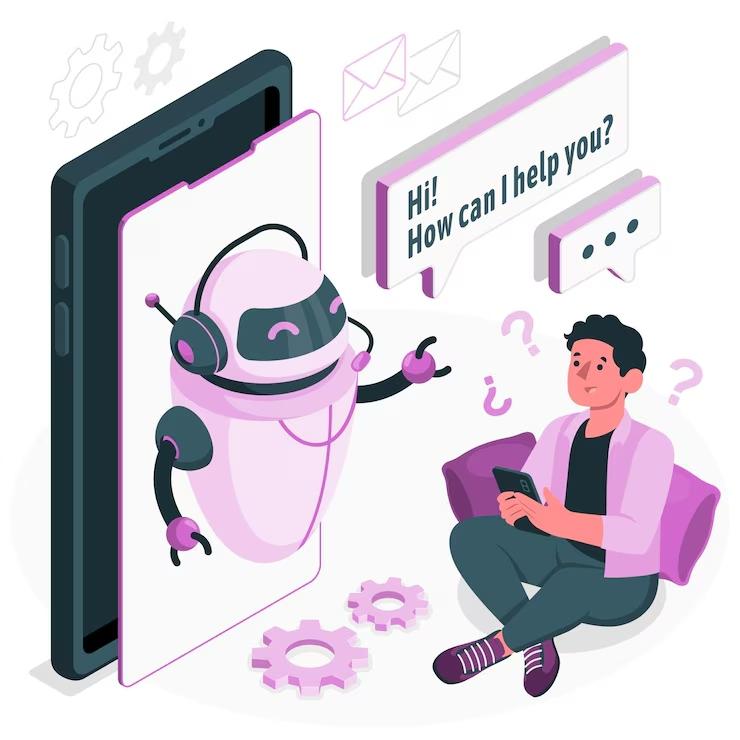
Before diving into code, consider the specific purpose of your AI chatbot. Some popular AI use cases include:
- Customer Support Chatbot: Assist customers with inquiries, troubleshooting, and product information.
- Recommendation Engine: Provide users with personalized suggestions based on their preferences.
- Personalized Assistant: Offer users guidance and tips, potentially as a coach or advisor in specific fields.
To narrow down your AI’s purpose, ask questions like: “Who is my target audience? What specific tasks or questions will my AI address?” This focus will help you structure the conversation and prioritize interactions.
Step 2: Structure Conversations for the Use Case
The structure of the conversation is critical in AI chatbots. Chai’s platform uses intents and responses to handle different aspects of a user conversation. Think of intents as the AI’s understanding of a user’s goal, such as greeting, asking a question, or saying goodbye.
For each use case, map out the main conversation flow. This typically includes setting up:
- Greeting Intent: Responds to initial user greetings.
- Primary Task Intent: Manages the AI’s core purpose, like handling support queries or making recommendations.
- Farewell Intent: Wraps up the conversation when the user is done.
Example: Creating a Customer Support Chatbot
In this example, we’ll create a basic customer support chatbot with the intent of greeting, query handling, and farewell.
Define Intents
Decide on the intents your chatbot will need, such as `greeting`, `query_support`, and `farewell`.
Build Dialog Flows
Write functions to handle each intent, allowing the AI to respond appropriately to user messages.
Example Code
“`python
from chai import ChaiClient
client = ChaiClient(api_key=”your_api_key”)
def handle_intent(intent, user_message):
if intent == “greeting”:
return “Hello! How can I assist you today?”
elif intent == “query_support”:
return “Please describe your issue, and I’ll do my best to help.”
elif intent == “farewell”:
return “Thank you for reaching out! Have a great day.”
else:
return “I’m here to help with any questions you have.”
Example usage
intent = “greeting”
response = handle_intent(intent, “Hi”)
print(response) # Output: “Hello! How can I assist you today?”
“`
This simple code structure allows you to build conversation flows that match different user intents, creating an engaging and helpful chatbot experience.
Training Your Chai AI
After coding the initial conversation flows, it’s time to train your AI to improve its responses. Training allows Chai to understand user inputs more accurately, especially as you gather data on common queries.
Data and Training Methods
- User Feedback
Track and analyze real interactions to identify common patterns or phrases that users use.
- Pre-built Datasets
Use existing datasets for common intents, such as greetings, common questions, and farewells.
- Custom Training Routines
Chai may offer tools for training, which can include providing multiple examples for each intent.
Example Code for Training
“`python
# Pseudo-code for training function
def train_bot(intents_data):
for intent, data in intents_data.items():
client.train_intent(intent, data[‘examples’], data[‘responses’])
By training the bot, you can create more sophisticated, personalized responses and adapt the bot’s language model to better handle the specific vocabulary or phrases relevant to your use case.
Testing and Iterating Your AI Application
Testing is a critical step before deploying your chatbot. By conducting various tests, you can verify that the bot handles all expected interactions smoothly.
Types of Testing
- Unit Testing
Test individual functions, such as `handle_intent`, to make sure they perform as expected.
- End-to-End Testing
Simulate user interactions from start to finish to check the full conversation flow.
- User Feedback Testing
Run a beta version and gather feedback from a small group of users. This can reveal unexpected issues or ways to improve the bot’s effectiveness.
Example: Test Script for Chai Chatbot
“`python
def test_chatbot():
assert handle_intent(“greeting”, “Hi”) == “Hello! How can I assist you today?”
assert handle_intent(“query_support”, “I need help with my order”) == “Please describe your issue, and I’ll do my best to help.”
print(“All tests passed!”)
test_chatbot()
“`
By iterating and improving based on testing outcomes, you can create a smoother, more reliable experience for users.
Deploying Your AI with Chai
Once testing is complete, you can deploy your Chai-powered chatbot. Chai provides options for publishing on its platform, or you can use its API to embed the AI into other websites or mobile apps.
- Deploy on Chai
Publish directly through Chai’s platform, making your chatbot accessible to users.
- Embed Using API
Integrate the chatbot into your existing website or app by embedding Chai’s API.
For instance, embedding the chatbot in a support page allows users to get real-time assistance without leaving the site. Deployment options will vary depending on the platform, but Chai provides flexible API tools for different needs.
Conclusion
How to code use cases for AI using Chai can streamline chatbot development for a variety of use cases, from customer support to personalized assistance. The Chai platform’s emphasis on conversation flows and NLP simplifies AI development, allowing you to focus on delivering valuable user interactions.
Whether you’re developing for a specific business need or just exploring conversational AI, Chai offers a robust toolset for quickly bringing your AI use case to life. For any assistance, Tambena Consulting is always here for you.
FAQs
How to make a good chai bot?
To make a good Chai bot:
- Define clear intents
- Create natural conversation flows
- Train with relevant data
- Test thoroughly for accurate and engaging responses.